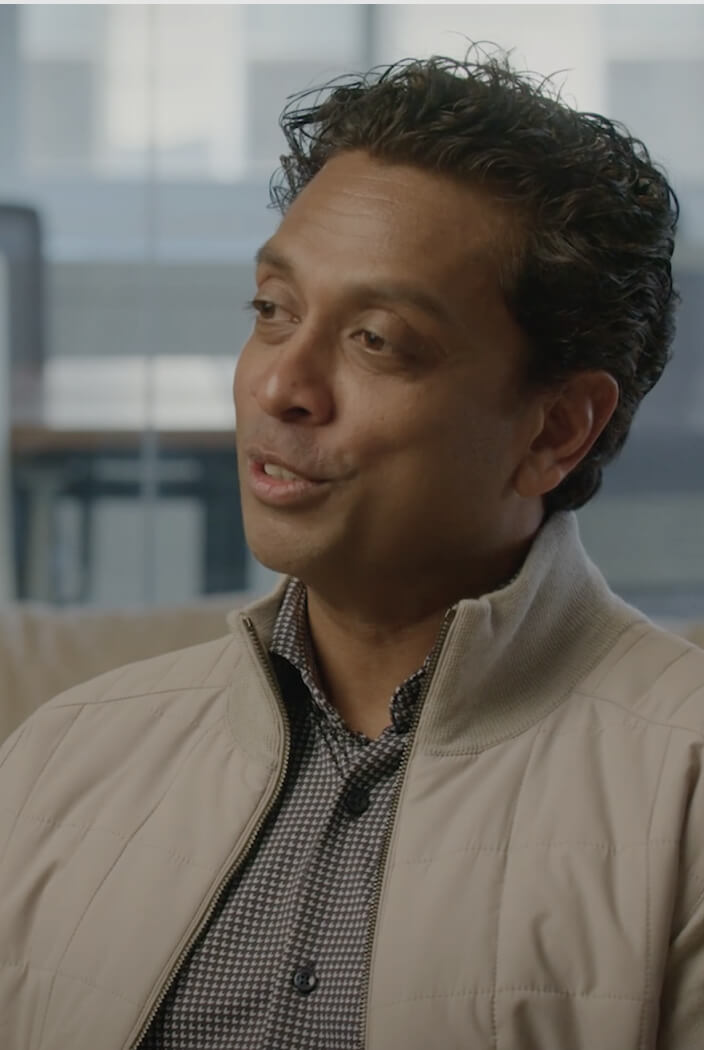
Most applications rely on a database, and CRUD is at the heart of that interaction. The acronym stands for the four basic data operations every app needs to manage data:
- Create
- Read
- Update
- Delete
If you’re working with an SQL database, almost every query you write falls into one of these four categories. Grasping them is the first step to building functional, data-driven apps.
In this article, we’ll cover:
- What CRUD means and why it’s important
- How to use SQL to perform each CRUD operation
- How to build a simple CRUD SQL interface
Let’s start with the CRUD definition.
What does CRUD stand for?
CRUD stands for Create, Read, Update, and Delete. These are the four basic operations used to manage data in a database:
- Create: Adds new records to a table. For example, inserting a new customer into a user database.
- Read: Retrieves existing data, like pulling up a list of products or showing user details.
- Update: Modifies existing records, such as changing the status of an order or editing a user’s email address.
- Delete: Removes records that are no longer needed, like clearing out old accounts or expired listings.
This CRUD model is the foundation of how most apps manage data behind the scenes.
CRUD SQL operations explained
Now that you know what CRUD stands for, let’s look at how each operation works in an SQL database. Each CRUD operation maps directly to common SQL commands and handles a specific part of the CRUD database lifecycle:
- Create → INSERT
Adds new records to a table.
INSERT INTO users (name, email) VALUES ('Alice', 'alice@example.com');
- Read → SELECT
Retrieves data from the database.
SELECT * FROM users WHERE email = 'alice@example.com';
- Update → UPDATE
Modifies existing records.
UPDATE users SET name = 'Alice Johnson' WHERE email = 'alice@example.com';
- Delete → DELETE
Removes records from the database
DELETE FROM users WHERE email = 'alice@example.com';
How to build a basic CRUD interface
A CRUD interface connects users to an SQL database, allowing them to manage records through forms, tables, and buttons rather than raw SQL queries.
Here’s how to build one step by step:
1. Define your use case
Before writing SQL or designing a UI, you need to understand what kind of data your application will manage. This step shapes your schema, queries, and interface, so it's worth spending a little time getting it right.
Start by identifying the core entity your CRUD interface will revolve around. That could be users, products, support tickets, or orders — whatever your app is designed to manage.
Once you’ve picked the entity, break it down further by listing the key pieces of information you’ll need to store. Think about which fields are required, which can be optional, and which need default values. For a users table, that might include their name and email address.
Each field should have a data type and, if needed, constraints. For example:
name VARCHAR(100) NOT NULL
email VARCHAR(255) NOT NULL UNIQUE
ensures no duplicate emailscreated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
tracks record creation
If your entity has relationships with other entities, map those early. For example:
- A ticket belongs to a user →
user_id
as a foreign key - A comment belongs to both a user and a post
Understanding relationships upfront will help you structure your schema using foreign keys and will also inform how your interface will behave (like showing a user’s name in a ticket view instead of just an ID).
2. Design the database schema
Once your use case is defined, it’s time to translate it into a working database structure.
Start by creating one table for each core entity. For every table, list out the fields (columns) you’ll need and assign an appropriate data type for each one based on the kind of data it will store.
A users table might look like this:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Every table should include a primary key to uniquely identify rows. If a table depends on another (like a ticket belonging to a user), you’ll need a foreign key as we mentioned earlier.
For example, the tickets table might reference users like this:
CREATE TABLE tickets (
id SERIAL PRIMARY KEY,
user_id INT NOT NULL REFERENCES users(id),
subject VARCHAR(255) NOT NULL,
status VARCHAR(50) DEFAULT 'open'
);
To improve performance, especially as your dataset grows, consider adding indexes on columns that are frequently used in `WHERE` clauses and joins. Common candidates
include:
- Foreign key fields (e.g.,
user_id
) - Fields used in searches or filters (e.g.,
email
,status
)
Just be mindful. Too many indexes can slow down inserts and updates, so index only where it adds value.
3. Set up the SQL database
Start by choosing your database system. Here are a few popular options:
- PostgreSQL: Highly regarded for its stability and features
- MySQL: Widely used, especially in LAMP stacks
- SQLite: Lightweight and file-based. Perfect for local testing, prototyping, or embedded apps
In this guide, we’ll use PostgreSQL for examples, but the same principles apply to other systems.
You’ll need either a local or cloud-based environment to run your database:
- Local development: Install Postgres using Homebrew, Docker, or your system’s package manager
- Cloud deployments: Use a managed platform like Supabase, Neon, Railway, or Amazon RDS to spin up an instance quickly
Once your database is set up, connect to it using a CLI like psql
or a GUI tool such as pgAdmin, DBeaver, or TablePlus.
- Local dev: Install Postgres or MySQL via Homebrew, Docker, or native installers.
- Cloud services: Use managed platforms like Supabase, Neon, Railway, or Amazon RDS to spin up a database instance.
Once installed or provisioned, connect to the database using psql or a GUI tool like pgAdmin, DBeaver, or TablePlus).
You can write raw SQL or use a migration tool to create your tables. Here’s an example in PostgreSQL:
CREATE DATABASE my_crud_app;
\c my_crud_app
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
You're now ready to start adding data and writing CRUD queries.
4. Seed sample data (Optional)
Seeding your database helps you test your CRUD interface before it’s connected to real inputs. It’s useful for mocking up the UI, testing edge cases, and validating query behavior across multiple rows.
You can seed data a few different ways:
Insert rows manually
Write INSERT INTO
statements for each fake record:
INSERT INTO
users (name, email) VALUES
('Alice Smith', 'alice@example.com'),
('Bob Jones', 'bob@example.com');
Import from a CSV or JSON file
For larger datasets, bulk importing data is more efficient. Most SQL engines support import functionality. PostgreSQL, specifically, provides the COPY command for this purpose:
COPY users(name, email)
FROM '/path/to/users.csv'
DELIMITER ','
CSV HEADER;
Use a script or ORM
If you’re using a backend language like Node.js or Python, you can write a script to seed the database using a library or ORM.
5. Write SQL Queries for CRUD
These queries are the core logic your UI will trigger behind the scenes.
Create
Use INSERT
to add new rows to your table
INSERT INTO users (name, email) VALUES ($1, $2);
Read
Use SELECT
to retrieve data from the table. This can be as simple or as complex as you need.
SELECT * FROM users ORDER BY created_at DESC;
You can add filters or ordering to support UI features like search, sort, or pagination:
SELECT * FROM users
WHERE status = 'active'
ORDER BY created_at DESC
LIMIT 10 OFFSET 0;
Update
UPDATE modifies existing data. Always scope it with a WHERE clause to avoid accidentally updating all rows.
UPDATE users SET name = $1 WHERE id = $2;
Delete
As with UPDATE
, always use WHERE
to avoid nuking the entire table.
DELETE FROM users WHERE id = $1;
For safer deletes and better auditability, consider using a status = 'deleted' flag instead of hard-deleting rows.
And always use parameterized queries to prevent SQL injection. Avoid injecting user input directly into your SQL strings.
6. Choose your interface method
There are multiple ways to build a CRUD interface, depending on how hands-on you want to be:
- Custom app: Build your own UI with HTML, JavaScript, and a backend like Node.js or Flask.
- BI tools with write-back: Use platforms like Metabase, Power BI, or Looker, especially when data editing is tied to reporting.
- Low-code tools: Platforms like Superblocks, Appsmith, or Budibase let you drag-and-drop components and bind them to your database. Great if shortened development cycles are part of your enterprise strategy.
- APIs: Wrap your SQL queries in REST or GraphQL endpoints for use across mobile or frontend apps.
- CLI: If it’s just for internal devs, a terminal-based interface may be all you need.
7. Build the user interface
Your interface should support all four CRUD actions:
- Create: A form to input new data (e.g., name, email)
- Read: A table or list view to display records
- Update: A form or modal that lets you edit a selected row
- Delete: A button or icon to remove a record
Design-wise, keep things clean and focused by:
- Using labels and placeholders for clarity
- Adding loading states and disabled buttons during query execution
- Grouping related actions (e.g., edit and delete buttons inside the table row)
If you're using a low-code tool, much of this wiring is handled for you. If you're building from scratch, libraries like React or Vue can make it easier.
8. Connect the UI to SQL operations
Once your interface is in place, each component needs to trigger the appropriate SQL query. This step involves wiring user actions like form submissions or button clicks to backend logic or endpoints that run your INSERT
, SELECT
, UPDATE
, and DELETE
statements.
9. Add input validation and error handling
Never trust user input. Validate both on the client and the server to make sure:
- Required fields are not empty
- Emails are in a valid format
- Nulls, unique constraint errors, or failed queries are handled gracefully
10. Secure the application
Even basic CRUD apps should follow enterprise-grade security practices. Since they interact with databases, a few minor oversights can lead to significant risks like unauthorized data access, SQL injection, or credential leaks.
Best practices include:
- Implementing Role-based access control (RBAC) to prevent data modification.
- Using parametrized queries to prevent SQL injection.
- Storing database credentials securely in environment variables or credential vaults.
11. Test the full CRUD flow
Before shipping anything, ensure each part of your CRUD interface works end to end:
- Can you add a record and see it show up?
- Does updating a record reflect changes in the UI?
- Are deleted records removed cleanly?
Test edge cases like duplicate values, missing fields, or SQL constraint errors. And finally, before calling it done, run your app against a fresh database without any seed data. This confirms that:
- Your schema creation scripts work
- Your interface handles empty states
- The app can bootstrap cleanly without relying on hardcoded data
12. Optimize for performance and scalability
Once things are working, make sure they scale. You can:
- Add indexes to speed up reads on frequently queried fields
- Paginate results instead of loading everything at once
- Use lazy loading or infinite scroll in the UI if needed
13. Document the application (Optional but recommended)
Docs are not required but are highly recommended. Create a short guide for developers or admins that explains:
- What tables and fields exist
- What each SQL query does
- How to modify the interface or add new fields
This makes handoffs easier and reduces future tech debt.
What tools help build CRUD interfaces?
There are a ton of tools that can help you build a CRUD interface. Here’s a quick overview of popular options:
- Low code and app builders: These are ideal for building CRUD apps quickly without writing much front-end or integration code. These tools are especially useful when you want a working interface fast but still need control over logic, queries, and access.
- Web frameworks (custom UI + backend): If you want full control and are comfortable coding, you can use a frontend library like React and connect to a backend API that runs your SQL queries.
- Database admin and query tools: These aren't full UI tools, but they make testing and building CRUD logic easier. For example, Hasura creates a GraphQL layer on top of a Postgres app based on your schema.
Frequently asked questions
What is the difference between CRUD and REST?
CRUD stands for Create, Read, Update, and Delete, which refers to the basic operations you perform on data. Conversely, REST is a web architecture style that uses standard HTTP methods such as POST, GET, PUT, and DELETE to expose those operations via APIs.
These REST APIs map directly to CRUD operations, like this:
POST /users
→ Create a new userGET /users/1
→ Read a userPUT /users/1
→ Update a userDELETE /users/1
→ Delete a user
Can you perform CRUD without SQL?
Yes. The concept of CRUD is universal. It applies to any system where you’re managing data, regardless of whether SQL is involved. You can perform CRUD operations on:
- NoSQL databases like MongoDB (using methods like
insertOne
,find
,updateOne
,deleteOne
) - Flat files or spreadsheets (manually or via scripts)
- APIs that abstract away the database entirely
Are CRUD operations secure?
They can be, but only if implemented carefully. By default, raw CRUD operations aren’t secure unless you add proper protections.
To secure CRUD operations, you should:
- Use parameterized queries to prevent SQL injection
- Enforce role-based access control so users can’t update or delete what they shouldn’t
- Sanitize and validate all inputs
- Store database credentials securely using environment variables or secret vaults
What is an example of a CRUD interface?
A user management dashboard is a classic CRUD interface. It typically includes:
- A form to add new users
- A table to view all users
- Edit buttons to update user details
- Delete buttons to remove users
What programming languages support CRUD operations?
Pretty much all modern languages support CRUD programming. Here are some common examples:
- JavaScript/TypeScript: With SQL libraries (
pg
,mysql2
) or NoSQL clients (MongoDB
,Firebase
) - Python: With ORMs like SQLAlchemy or direct SQL via
psycopg2
- Java: Using JDBC, Spring Data, or Hibernate
- Ruby: With ActiveRecord in Ruby on Rails
How Superblocks helps citizens developers build
Superblocks is a great tool for building CRUD interfaces without setting up the frontend, backend, and data integrations from scratch. You get full flexibility to write raw SQL, direct access to your database, and a visual interface for binding those queries to forms, tables, buttons, and more.
This is thanks to our comprehensive set of features:
- Custom SQL with variables: Write raw SQL, bind it to UI components, and pass dynamic values directly from forms, tables, or filters.
- Fast, flexible delivery with AI building: Speed up the development of your interfaces with AI-assisted code generation, visual builders, and pre-built components.
- AI-generated mock data for prototyping: Use AI to generate example SQL queries and mock responses for testing and prototyping your interfaces — helpful when you're building without real data.
- 60+ native integrations: Easily connect your SQL database and extend your CRUD interface with data from other tools like Salesforce, S3, Zendesk, or internal APIs with pre-built connectors.
- Scoped access and role-based security: Limit actions (like update or delete) based on the current user's role or ownership.
- Developer experience: Build with real code, version changes with Git and integrate with your CI/CD pipelines.
- Built-in dev/prod environments: Test your CRUD logic against a staging DB, then deploy it to prod with a click.
- Audit-ready logs: Every query and action is logged for traceability and compliance critical for CRUD interfaces that touch production data.
- No vendor lock-in: You can self-host with the on-prem agent, keep data in your infrastructure, and export your app logic at any time.
- Component logic and conditional UI: Dynamically show or hide form fields, disable buttons, or display error messages based on query results or user input.
If you’d like to experience these features in action, check out our 5-minute Quickstart guide, or better yet, try Superblocks for free.
Stay tuned for updates
Get the latest Superblocks news and internal tooling market insights.
Table of Contents